✔ Unity - Mysql, 마리아 DB 연동하기
mysql dll import , mysql select, update, insert
MS-SQL은 유니티에 내장되어있어서 쓰기가 편한데 ..
Mysql, Maria DB는 별도 DLL을 구해서 사용해야되니까 불편하당 ㅠ_ㅠ
유니티에서 Mysql 혹은 MariaDB를 연동해야할때는 Mysql dll을 넣어줘야합니다.
마리아 DB도 Mysql dll로 같이 가능합니다 ㅎㅎ 1타 쌍피..
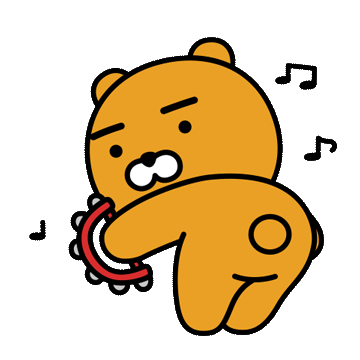
혹시 서버를 먼저 구축해야한다면 아래 포스트를 참조하여 서버 구축을 해주세요~
2023.03.22 - [DB] - [MariaDB] 윈도우에서 MariaDB 서버 구축 및 연결하기
[MariaDB] 윈도우에서 MariaDB 서버 구축 및 연결하기
✔ Window OS Maria DB install and connect ● 마리아DB 서버 설치하기 https://mariadb.org/ MariaDB Foundation - MariaDB.org … Continue reading "MariaDB Foundation" mariadb.org 홈페이지에서 다운로드 탭 클릭! 자신의 컴퓨터에
timeboxstory.tistory.com
◈ 유니티 Mysql, Maria DB 사용하기 위한 DLL 임포트
mysql Connector/Net 다운로드 페이지
https://downloads.mysql.com/archives/c-net/
MySQL :: Download MySQL Connector/NET (Archived Versions)
Please note that these are old versions. New releases will have recent bug fixes and features! To download the latest release of MySQL Connector/NET, please visit MySQL Downloads. MySQL open source software is provided under the GPL License.
downloads.mysql.com
Connector 버전은 6.9.5 이하버전으로 다운로드 하셔야합니다. (최신버전은 유니티에서 지원이 안됨)
.NET & Mono를 선택하여 다운로드를 합니다.
다운로드 한뒤에 설치를 완료되면 DLL을 찾아야합니다.
설치경로 : C:\Program Files (x86) \ MySQL \ MySQL Connector Net 버전 \ Assemblies
여기 들어가면 .net 버전별로 폴더가 나타나고 폴더안에는 MySql.Data.dll이 있습니다.
유니티 프로젝트의 Plugins 폴더를 만들어 넣어줍니다.
혹시 아래와 같은 에러가 나시나요?
Assembly 'Assets/Plugins/MySql.Data.dll' will not be loaded due to errors: Unable to resolve reference 'Google.Protobuf'. Is the assembly missing or incompatible |
위 오류는 위에서 언급했던 것과 같이 Mysql DLL이 높은 버전으로 유니티에서 호환되지 않습니다.
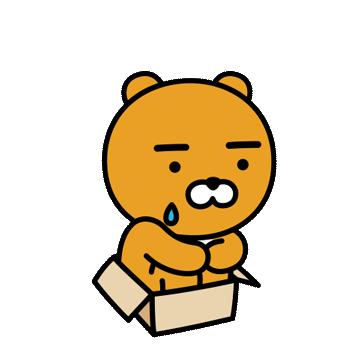
잘 안되시면 아래에 첨부된 제가 사용하는 Mysql DLL 다운로드 하세요 ( 유니티 버전 테스트 : 2020.2.1f 잘됨 )
다운로드한 DLL을 유니티 프로젝트의 Plugins 폴더에 넣습니다 (없으면 생성)
이제 에러가 안나시겠죠!?
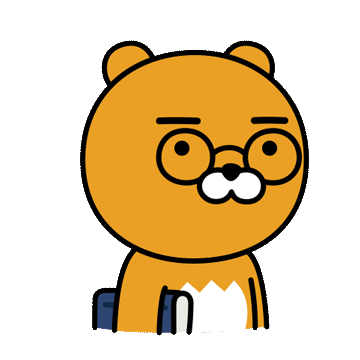
◈ 유니티에서 접속 및 쿼리 조회 방법
이제 유니티에서 Mysql, Maria DB 어떻게 연결해서 사용되는지 아래 예제 코드를 참고해주세요~
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.Data;
using MySql.Data.MySqlClient;
public class DB_Control : MonoBehaviour
{
public static MySqlConnection SqlConn;
static string ipAddress = "127.0.0.1";
static string db_id = "userid";
static string db_pw = "1234";
static string db_name = "DabaseName";
string strConn = string.Format("server={0};uid={1};pwd={2};database={3};charset=utf8 ;", ipAddress, db_id, db_pw, db_name);
private void Awake()
{
try
{
SqlConn = new MySqlConnection(strConn);
}
catch (System.Exception e)
{
Debug.Log(e.ToString());
}
}
void Start()
{
//Select TEST
string query = "select * from TB_TABLE";
DataSet ds = OnSelectRequest(query, "TB_TABLE");
Debug.Log(ds.GetXml());
}
//데이터 삽입,업데이트 쿼리시 사용 함수
public static bool OnInsertOrUpdateRequest(string str_query)
{
try
{
MySqlCommand sqlCommand = new MySqlCommand();
sqlCommand.Connection = SqlConn;
sqlCommand.CommandText = str_query;
SqlConn.Open();
sqlCommand.ExecuteNonQuery();
SqlConn.Close();
return true;
}
catch (System.Exception e)
{
Debug.Log(e.ToString());
return false;
}
}
//select 조회 쿼리시 사용
//2번째 파라미터 table_name은 Dataset 이름을 정의하기 위함
public static DataSet OnSelectRequest(string p_query, string table_name)
{
try
{
SqlConn.Open(); //DB 연결
MySqlCommand cmd = new MySqlCommand();
cmd.Connection = SqlConn;
cmd.CommandText = p_query;
MySqlDataAdapter sd = new MySqlDataAdapter(cmd);
DataSet ds = new DataSet();
sd.Fill(ds, table_name);
SqlConn.Close(); //DB 연결 해제
return ds;
}
catch (System.Exception e)
{
Debug.Log(e.ToString());
return null;
}
}
private void OnApplicationQuit()
{
SqlConn.Close();
}
}
쿼리 작성 방법은 다른 시스템과 별 차이가 없으니 아래 포스트를 참고해주세요
2019.09.26 - [Programming/C#] - [C#] MSSQL 접속 , 데이터 조회(select), 삽입(insert), 업데이트(update) 쿼리
[C#] MSSQL 접속 , 데이터 조회(select), 삽입(insert), 업데이트(update) 쿼리
✔ C# MSSQL 연결 select, insert, update 쿼리 예제 접속 문자열(ConnectionSting) 설정 Data Source : 연결할 주소 Initial Catalog : 초기 데이터 베이스 이름 Windows 인증 사용예 string strConn = "Data Sou..
timeboxstory.tistory.com
코드는 생각보다 간단하죠? ㅎㅎ
감사합니다 !!
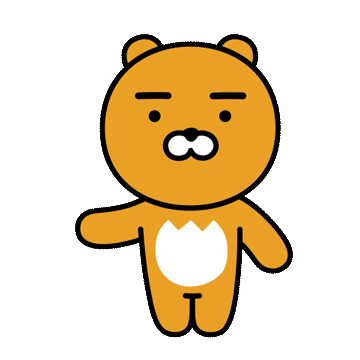
'Programming > Unity' 카테고리의 다른 글
[Unity/C#] 엑셀 파일 불러오기 (.xlsx) (3) | 2022.12.05 |
---|---|
[Unity] 유니티 컴파일 에러 - Assembly 'unityplastic' , PlasticSCM 관련 (2) | 2022.10.05 |
[Unity, C#] Serialize를 활용하여 데이터를 파일로 저장하기 (List, Dictionary, Array, Class) (2) | 2022.09.22 |
[Unity] 충돌호출 함수 OnTrigger와 OnCollision 사용법 및 차이 (0) | 2022.09.15 |
[Unity] [해결] WebGL 빌드 시 나타나는 오류 - IL2CPP (2) | 2022.06.22 |
댓글